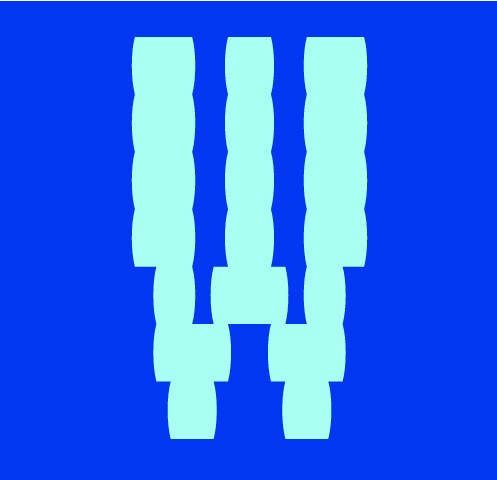
Iterating Over Elements In Nightwatch
Nightwatch.js is a powerful testing framework that allows you to easily automate browser testing. One of the challenges of browser testing is testing multiple identical items on a page. In this article, we will explore how to use Nightwatch to test dozens of identical items on a page.
Before we begin, it's important to note that you will need to have Nightwatch installed on your system. You can do this by running the following command:
npm install nightwatch
When dealing with dozens of identical items on a page, it's important to use the appropriate Nightwatch commands to select and interact with the elements. The most commonly used commands for this purpose are .elements() and .elementId().
The .elements() command is used to select multiple elements by their CSS selector. For example, if we want to select all the button elements on a page, we would use the following command:
browser.elements('css selector', 'button', function(result) {
// do something with the result
});
The .elementId() command is used to select a single element by its ID. For example, if we want to select a button with the ID of submit-button, we would use the following command:
browser.elementId('submit-button', function(result) {
// do something with the result
});
Once we have selected the elements, we can use a variety of Nightwatch commands to interact with them. For example, we can use the .click() command to click on a button, or the .setValue() command to set the value of an input field.
Here's an example of how you might use Nightwatch to test a page with dozens of identical items:
module.exports = {
'Test identical items': function (browser) {
browser
.url('http://example.com')
.waitForElementVisible('body', 1000)
.elements('css selector', 'button', function(result) {
result.value.forEach(function(element) {
browser.elementIdClick(element.ELEMENT);
});
})
.end();
}
};
This test will navigate to the website's home page, wait for the body element to be visible, then select all the button elements using the .elements() command, and then iterate through each element and clicking on each button using the .elementIdClick() command.
In order to run the test, you can use the following command:
nightwatch tests/identical_items_test.js
This will run the identical_items_test.js file and output the results to the console.
In conclusion, Nightwatch.js is a powerful tool for automating browser testing and can be used to easily test dozens of identical items on a page by using the appropriate commands to select and interact with the elements. With the help of this example, you can create your own tests to suit your specific needs and ensure that your application is working as expected.
More Posts
Blocking Ad Traffic In Nightwatch JS
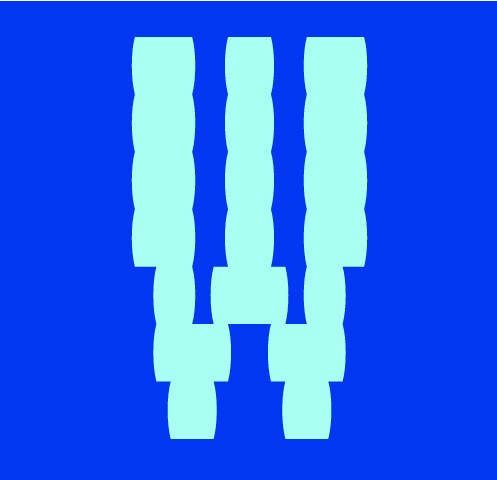
Example showing how you can block unwanted ad traffic in your Nightwatch JS tests....
Blocking Ad Traffic In Cypress
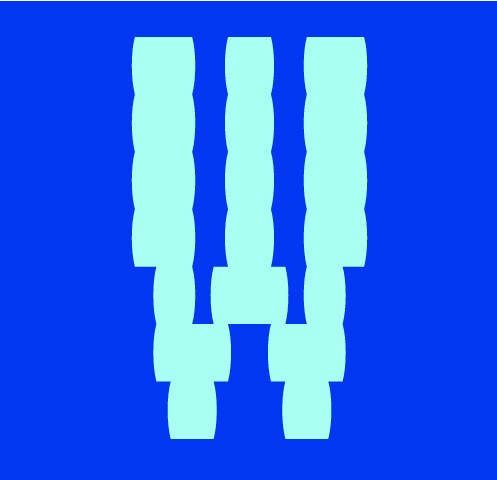
Example showing how you can block unwanted ad traffic in your Cypress tests....
Three Ways To Resize The Browser In Nightwatch
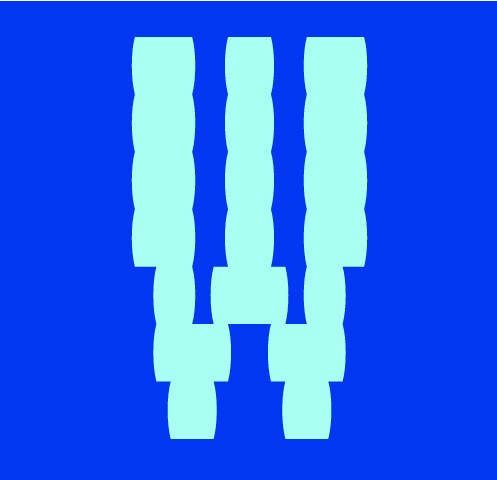
Outlining the three different ways to resize the browser in Nightwatch JS with examples....
Happy Path VS Sad Path Testing
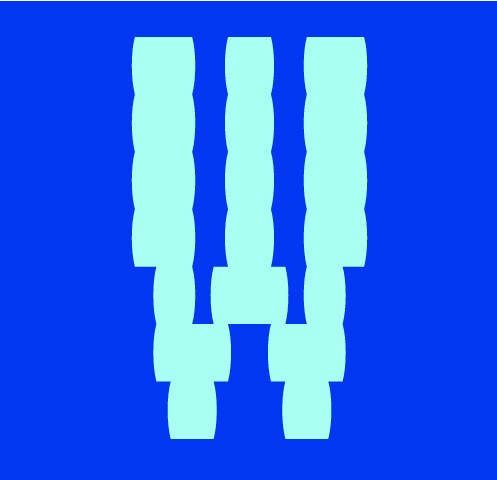
As a test engineer it is crucial that both happy path and sad path use cases have been considered and fully tested...